Contents
Outline
In this blog post, I will introduce how to provide multiple languages for the localization by GetX in Flutter. You can see full source code of this blog post on the link below.
Blog series
This blog post is made in the series about how to use GetX in Flutter. If you want to see the other features of the GetX, please check out the following blog posts.
- [GetX] State management
- [GetX] Route management
- [GetX] Dependency management
- [GetX] Localization
- [GetX] Theme
- [GetX] BottomSheet
- [GetX] Dialog
- [GetX] Snackbar
- [GetX] Platform and device info
GetX installation
To check how to use GetX for the localization, execute the command below to create a new Flutter project.
flutter create localization
And then, execute the command below to install the GetX
package.
flutter pub add get
Now, let’s see how to use GetX for the localization.
Create Translations
To use GetX for supporting multiple languages, we need to create a class inherited Translations
. Create lib/languages.dart
file and modify it like the below.
import 'package:get/get.dart';
class Languages extends Translations {
@override
Map<String, Map<String, String>> get keys => {
'ko_KR': {
'greeting': '안녕하세요',
},
'ja_JP': {
'greeting': 'こんにちは',
},
'en_US': {
'greeting': 'Hello',
},
};
}
To provide multiple languages, we need to set messages by key-value
in the country-language code.
Configure Translations
Next, we need to configure the Translations
class for that GetX can use it. Open the lib/main.dart
file and configure the Translations
class to GetMaterialApp
like the below.
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'langauges.dart';
...
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return GetMaterialApp(
translations: Languages(),
locale: Get.deviceLocale,
fallbackLocale: const Locale('en', 'US'),
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
You can see that the Translations
class is configured to GetMaterialApp
.
GetMaterialApp(
translations: Languages(),
...
);
ANd then, configure the locale
option to initial the language.
GetMaterialApp(
...
locale: Get.deviceLocale,
...
);
In here, we’ve used the Get.deviceLocale
of GetX to get the device language.
GetMaterialApp(
...
fallbackLocale: const Locale('en', 'US'),
...
);
However, we don’t provide all languages, so if the device language is not supported, we need to show the default langauge. For this, we’ve configured the fallbackLocale
for the default language.
Use Translations
Next, let’s see how to use the localized messages. Open the lib/main.dart
file and modify it like the below.
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'greeting'.tr,
style: Theme.of(context).textTheme.headline4,
),
OutlinedButton(
onPressed: () => Get.updateLocale(const Locale('ko', 'KR')),
child: const Text('Korean'),
),
],
),
),
);
}
}
To show the localized message, you can use the tr
of GetX like the below.
'greeting'.tr,
You can use it in the screen(build) and also, you can use it anywhere.
If you want to change the language, you can use the Get.updateLocale
of GetX like the below.
Get.updateLocale(const Locale('ko', 'KR'))
Check
When you execute the code, you can see the localized message depends on the device.
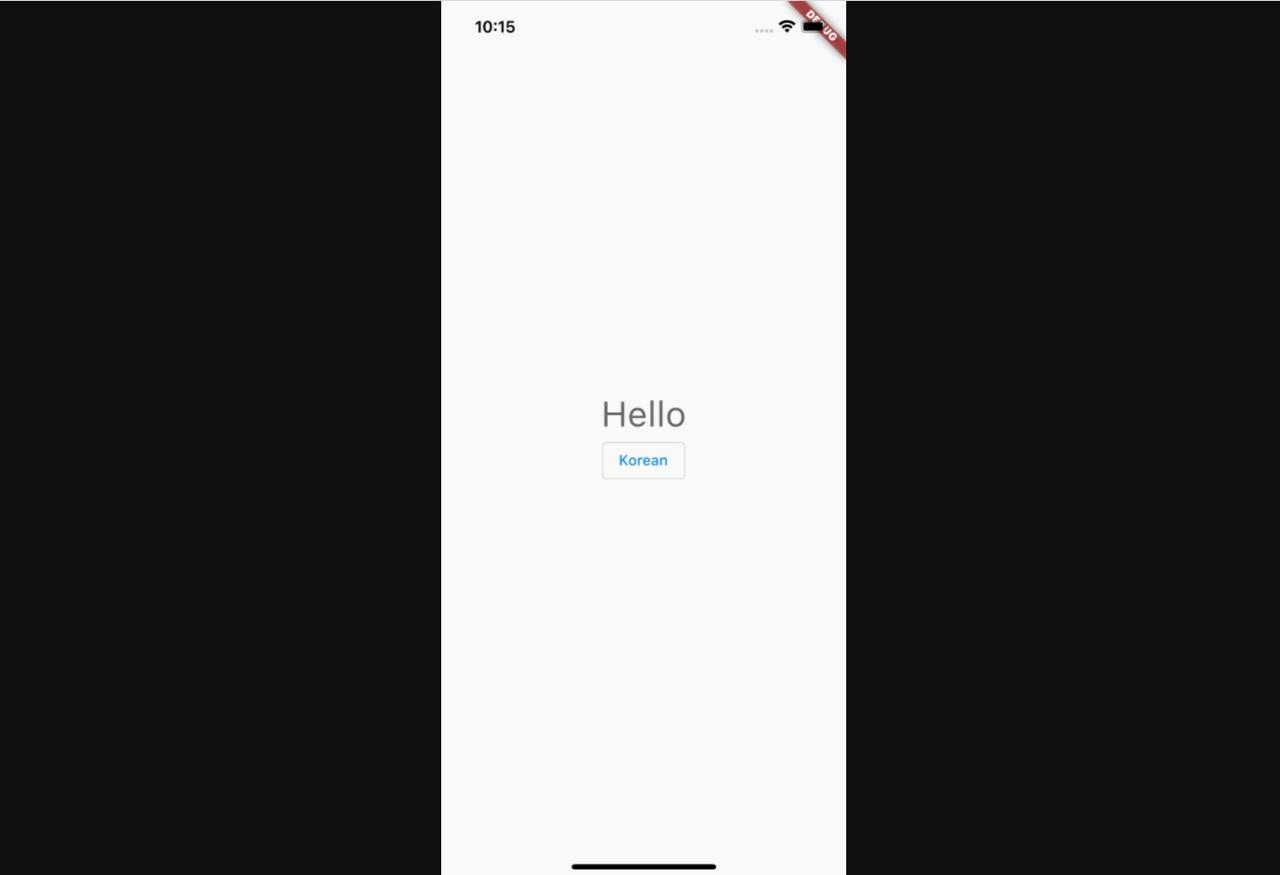
Next, when you press the button on the screen to change the Korean message, you can see the Korean message is shown well.
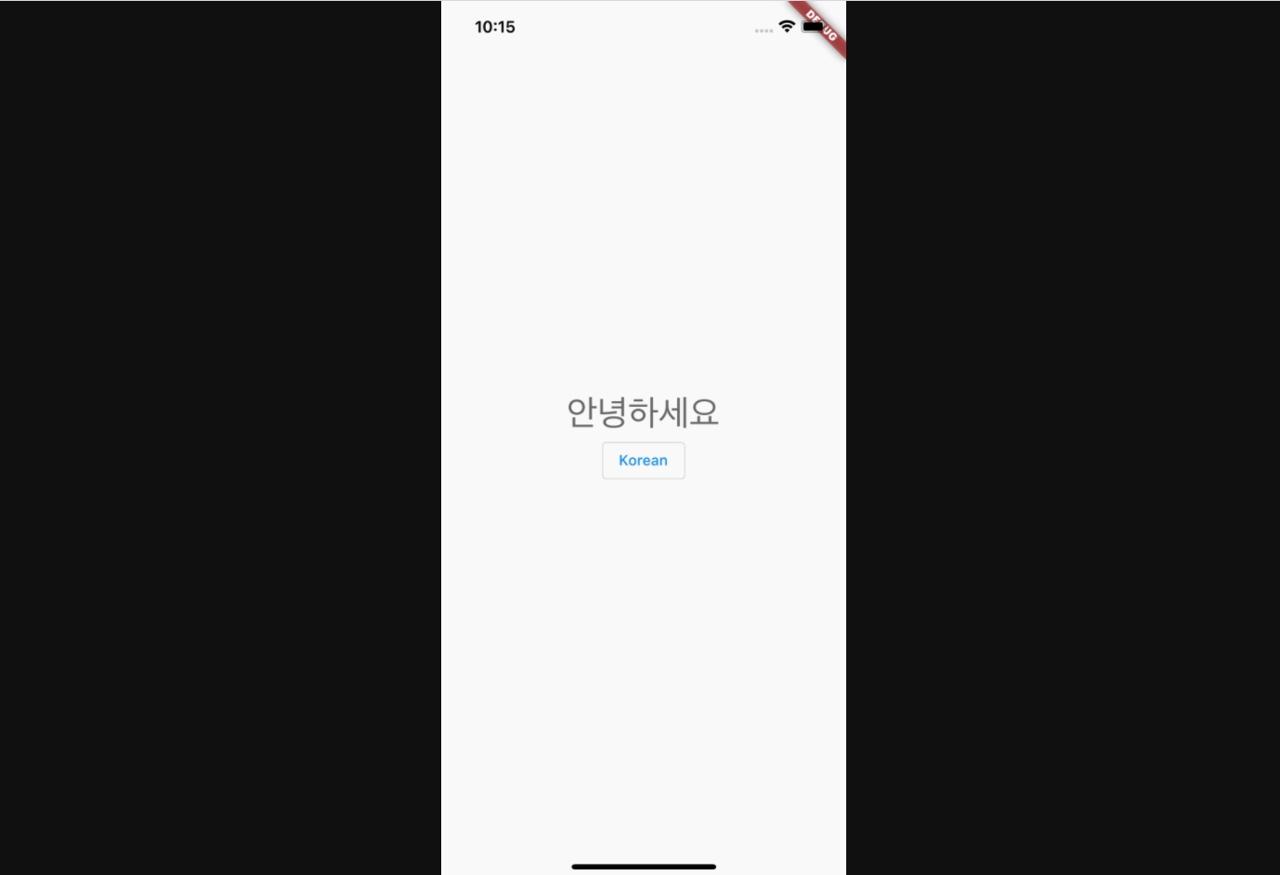
Complted
Done! we’ve seen how to use GetX to provide the localized messages in Flutter. If you don’t want to use GetX or GetX localization feature, you can refer the Flutter official document for supporting the localization.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.