Outline
In this blog post, I will introduce how to use in_app_review to show the app review dialog that the user can write the review in the app.
- in_app_review: https://pub.dev/packages/in_app_review
You can see the full source code of this blog post in the following link.
Install in_app_review
To check how to use in_app_review in Flutter, execute the following command to create a new Flutter project.
flutter create in_app_review_example
And then, execute the following command to install the in_app_review
package.
flutter pub add in_app_review
Next, let’s see how to use the in_app_review
package.
How to use
To use in_app_review
is very simple. You can show the app review dialog in the app by using the in_app_review
package like the below.
import 'package:in_app_review/in_app_review.dart';
final InAppReview inAppReview = InAppReview.instance;
if (await inAppReview.isAvailable()) {
inAppReview.requestReview();
}
Example
To check how to use in_app_review, open the lib/main.dart
file and modify it like the below.
import 'package:flutter/material.dart';
import 'package:in_app_review/in_app_review.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('In App Review Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () async {
final InAppReview inAppReview = InAppReview.instance;
if (await inAppReview.isAvailable()) {
inAppReview.requestReview();
}
},
child: const Text('Open App Review'),
),
],
),
),
);
}
}
When you execute the code, you can see the Open App Review
button on the center of the screen like the below.
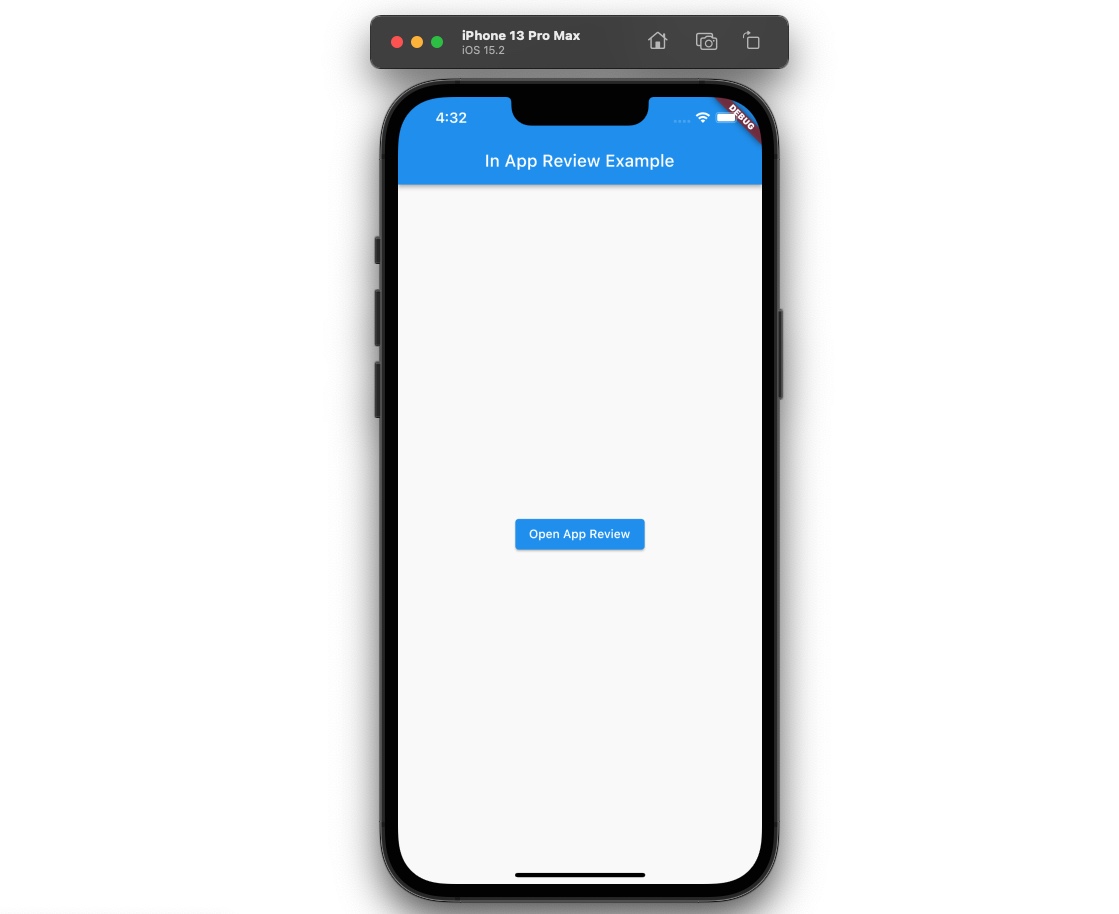
And then, when you press the button, the app review dialog will be shown by the following in_app_review
code.
final InAppReview inAppReview = InAppReview.instance;
if (await inAppReview.isAvailable()) {
inAppReview.requestReview();
}
If you don’t have any problem, you can see the app review dialog like the below. (If you can’t see it on the simulator, try it on the real device.)
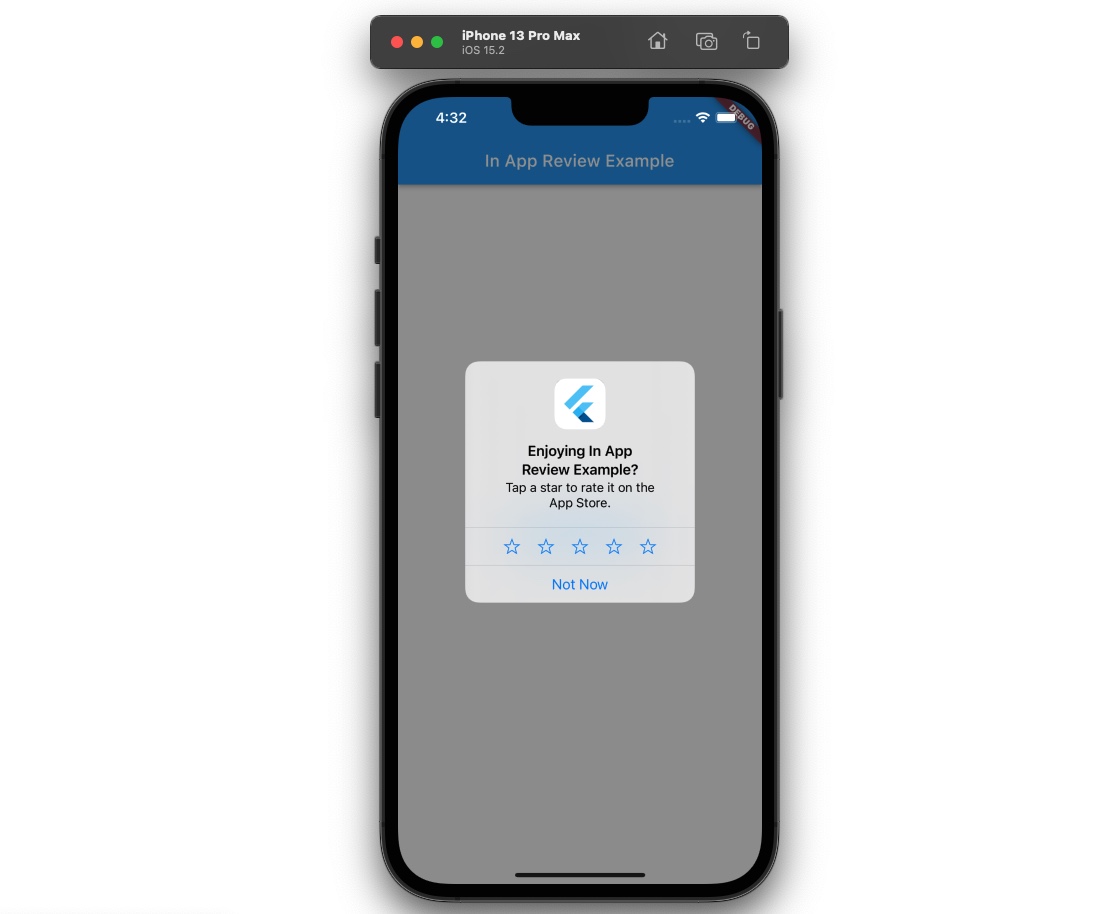
Completed
Done! we’ve seen how to use in_app_review
to show the app review dialog in the app in Flutter, Now, you can try to use in_app_review to show the app review dialog in the app, and make the user can write the app review more simply.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.