Contents
Outline
In the previous blog post, I introduced how to use webview_flutter to show the web page in the app.
In this blog post, I will introduce how to use cookie with webview_flutter.
You can see the full source code of this blog post on the following link.
- GitHub: https://github.com/dev-yakuza/study-flutter/tree/main/packages/webview_flutter_cookie_example
Create Flutter project
To check how to use webview_flutter to show the web page and manage the cookie in Flutter, execute the following command to create a new Flutter project.
flutter create webview_flutter_cookie_example
Install webview_flutter
To use webview_flutter
, execute the following command to insta the webview_flutter
package.
# cd webview_flutter_cookie_example
flutter pub add webview_flutter
Android configuration
To use webview_flutter
in Android, you need to configure 19
to minSdkVersion
. Open the ./android/app/build.gradle
file and modify it like the below.
android {
defaultConfig {
minSdkVersion 19
}
}
Show web page
To show the web page by webview_flutter
, open the ./lib/main.dart
file and modify it like the below.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
initialUrl: 'https://deku.posstree.com/en/',
javascriptMode: JavascriptMode.unrestricted,
),
);
}
}
When you execute the code, you can see the screen like the below.
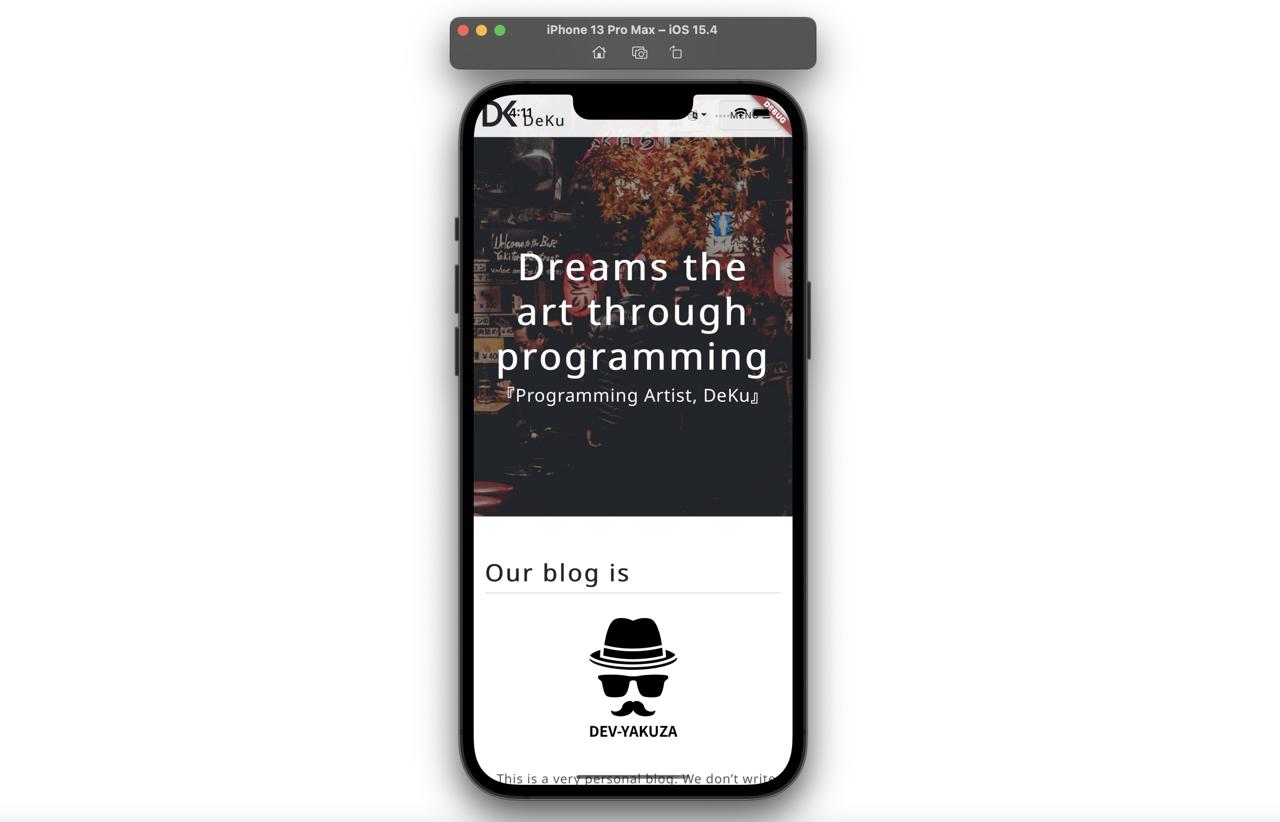
Get cookie
The webview_flutter
package doesn’t support to get the cookie basically. So, we need to use the runJavascriptReturningResult()
function of WebViewController
to execute the JavaScript code to get the cookie.
First, create a WebViewController
instance like the below.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class _MyHomePageState extends State<MyHomePage> {
late WebViewController _webViewController;
...
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
...
onWebViewCreated: (WebViewController webViewController) {
_webViewController = webViewController;
},
),
);
}
}
And then, create the floatingActionButton
button like the below. And, get the cookie and show it when the button is tapped.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class _MyHomePageState extends State<MyHomePage> {
...
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
...
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
final cookies = await _webViewController.runJavascriptReturningResult(
'document.cookie',
);
print(cookies);
},
child: const Icon(Icons.web_outlined),
),
);
}
}
When you execute the code, you can see the following screen.
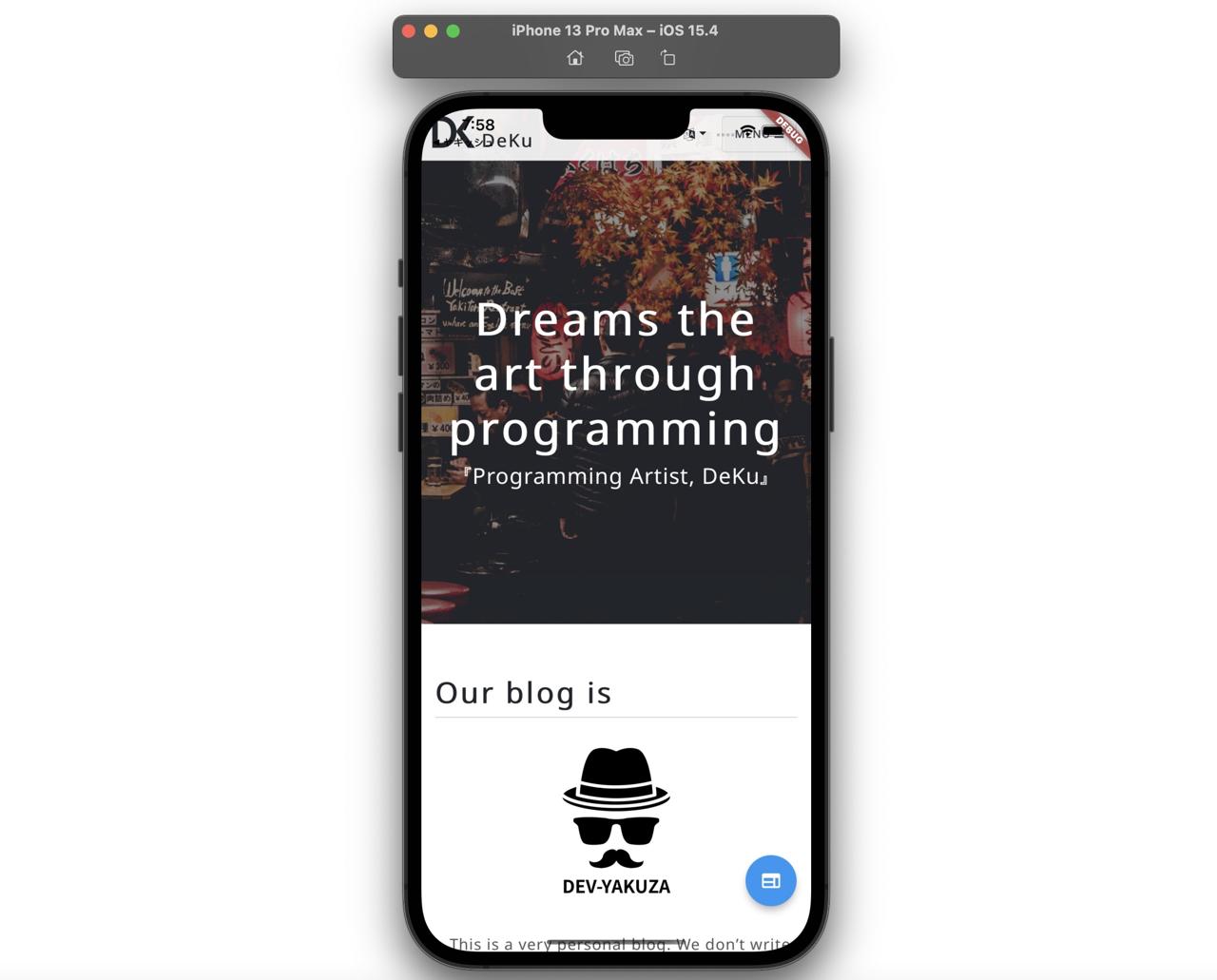
And, when you tap the button, you can see the the cookie is shown in Debug console
like the below..
flutter: GoogleAdServingTest=Good; __gads=ID=ab7b82a700ee6e5a-22c083926ed200a5:T=1650798117:RT=1650798117:S=ALNI_MbIWGRfzm5ErMMkuSLP0aMebyo_Wg; _ga=GA1.2.722169585.1650798118; _gat_gtag_UA_125408913_1=1; _gid=GA1.2.746873769.1650798118
Delete specific cookie
The webview_flutter
package basicaaly doesn’t support to delete the specific cookie. So, you need to use the runJavascriptReturningResult()
function of WebViewController
to execute the JavaScript code to delete the specific cookie.
To check it, modify the floatingActionButton
button like the below.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class _MyHomePageState extends State<MyHomePage> {
...
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
...
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
var cookies = await _webViewController.runJavascriptReturningResult(
'document.cookie',
);
print(cookies);
await _webViewController.runJavascriptReturningResult(
'document.cookie = "_ga=; expires=Thu, 01 Jan 1970 00:00:00 UTC; domain=.posstree.com; path=/;"',
);
cookies = await _webViewController.runJavascriptReturningResult(
'document.cookie',
);
print(cookies);
},
child: const Icon(Icons.web_outlined),
),
);
}
}
And, when you tapped the button again, you can see the _ga
cookie is deleted well in the Debug console
like the below.
...
flutter: _gat_gtag_UA_125408913_1=1; _gid=GA1.2.746873769.1650798118; __gads=ID=ab7b82a700ee6e5a-22c083926ed200a5:T=1650798117:RT=1650798117:S=ALNI_MbIWGRfzm5ErMMkuSLP0aMebyo_Wg
CookieManager
The webview_flutter
provide the CookieManager
class to manage the cookie. When you use it, you can set the cookie and delete all cookies.
Delete all cookies
To delete all cookies with CookieManager
, create a CookieManager
instance like the below first.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class _MyHomePageState extends State<MyHomePage> {
late WebViewController _webViewController;
final _cookieManager = CookieManager();
...
}
To delete all cookies, you need to call the clearCookies()
function of CookieManager
. To check it, modify the floatingActionButton
button like the below.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class _MyHomePageState extends State<MyHomePage> {
...
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
...
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
...
await _cookieManager.clearCookies();
cookies = await _webViewController.runJavascriptReturningResult(
'document.cookie',
);
print(cookies);
},
child: const Icon(Icons.web_outlined),
),
);
}
}
And, when you tapped the button again, you can see all cookies are deleted well in the Debug console
like the below.
...
flutter:
Set cookie
You can set the value to the specific cookie by calling the setCookie()
function of CookieManager
. To check it, modify the floatingActionButton
button like the below.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
...
class _MyHomePageState extends State<MyHomePage> {
...
@override
Widget build(BuildContext context) {
return Scaffold(
body: WebView(
...
),
floatingActionButton: FloatingActionButton(
onPressed: () async {
...
await _cookieManager.setCookie(
const WebViewCookie(
name: "test",
value: "value",
domain: ".posstree.com",
),
);
cookies = await _webViewController.runJavascriptReturningResult(
'document.cookie',
);
print(cookies);
},
child: const Icon(Icons.web_outlined),
),
);
}
}
Now, when you tapped the button, you can see the cookie is set well in the Debug console
like the below.
...
flutter: test=value
Complated
Done! we’ve seen how to use webview_flutter
to show the web page in the app, and how to get or delete the cookie of the web site in Flutter. Also, we’ve seen how to use CookieManager
to delete all cookies or set the value to the specific cookie.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.