Contents
Outline
In this blog post, I will introduce what Package
is and how to use Package
in Golang. You can see the full source code of this blog post on the link below.
Package
Package is a basic unit to bind the codes in Golang. So, all codes in Golang should be in the package.
Also, when you write the codes in Golang, the main
package should be, and the main
function should be defined in the main
package. In Golang, the main function in the main package is an entry point of the program.
Other packages than the main package doesn’t include the entry point(main function), and are the sub-packages of the main package.
And the module has all packages. If you want to know the details about the module, you can see the link below.
How to use package
To check how to use Package, create the main.go
file and modify it like the below.
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println(rand.Int())
}
In this example, the main
package loads the fmt
package and the rand
package under the math
package to display the random number in the screen. When you execute the code above, you can see the following result.
# go run main.
5577006791947779410
Pulbic package
You can make the type, global variables, constants, functions, and methods to the public for using them in other packages. To make them public, the name should start with a capital letter, and if you don’t want to share them, the name should start with a lowercase letter.
To share the package and use the public package, you should use the module. If you want to know the details about the module, you can see the link below.
To make the public package, create the greeting
folder and the greeting.go
file in the folder, and modify it like the below.
package greeting
import "fmt"
const PI = 3.14
func PrintGreeting() {
print()
}
func print() {
fmt.Println("Hello, World!")
}
When you modify like the above, execute the following command to create the greeting
module.
go mod init github.com/dev-yakuza/study-golang/package/greeting
This greeting
package will be shared via the greeting
module. Next, let’s create the main
pakcage to use the greeting
package.
Create the main
folder in the same location with the greeting
folder, and then create the main.go
file and modify it like the below.
package main
import (
"fmt"
"github.com/dev-yakuza/study-golang/package/greeting"
)
func main() {
fmt.Println(greeting.PI)
greeting.PrintGreeting()
}
Next, execute the command below to create the module.
go mod init github.com/dev-yakuza/study-golang/package/main
After creating the module, execute the command below to use the greeting
module in the local.
go mod edit -replace github.com/dev-yakuza/study-golang/package/greeting=../greeting
Lastly, execute the following command to finish the module configuration.
go mod tidy
And then, when you execute the program that we’ve made, you can see the result like the below.
# go run main.go
3.14
Hello, World!
init function
The package can have the init
function, and this init
function is called once when the package is imported. This init
function is normarlly used for initializing the global variables in the package.
To check this, modify the greeting/greeting.go
file like the below.
package greeting
import "fmt"
const PI = 3.14
var globalVal = 0
func init() {
fmt.Println("init()", globalVal)
globalVal++
}
func PrintGreeting() {
print()
}
func print() {
fmt.Println("Hello, World!", globalVal)
}
And execute the following command in the main
folder to check the modification.
go run main.go
When the program is executed, you can see the following result.
init() 0
3.14
Hello, World! 1
Alias package name
In Golang, you can write programs with the packages. However, not all developers promise to create packages, so sometimes the package name is duplicated.
import (
"text/template"
"html/template"
)
template.New("foo").Parse(`{{define "T"}}Hello{{end}}`)
template.New("foo").Parse(`{{define "T"}}Hello{{end}}`)
When you use the duplicated package name like this, you can use Alias Package Name
.
import (
htmlplate "html/template"
"text/template"
)
template.New("foo").Parse(`{{define "T"}}Hello{{end}}`)
htmlplate.New("foo").Parse(`{{define "T"}}Hello{{end}}`)
To check this, modify the main.go
file like the below.
package main
import (
"fmt"
htmlplate "html/template"
"text/template"
)
func main() {
fmt.Println(template.New("foo").Parse(`{{define "T"}}Hello{{end}}`))
fmt.Println(htmlplate.New("foo").Parse(`{{define "T"}}Hello{{end}}`))
}
When you execute the program, you can see the following result.
# go run main.go
&{foo 0xc00010e480 0xc00006c050 } <nil>
&{<nil> 0xc000104140 0xc00010e900 0xc00006c0a0} <nil>
Blank identifier
In Golang, when you import the package, the package should be used like the defined variables. If you don’t use it, the compile error occurs. However, sometimes, we need to import the package only and not use it for calling the init()
function to initialize the global variables in package. At this time, you can use Alias package name
and Bacnk identifier(_)
to solve this problem.
import (
"database/sql"
_ "github.com/mattn/go-sqlite3"
)
When the package is imported, the init()
function is called basically. In other words, you need to call the init()
function by importing the package but you don’t need to use the other features, you can use the alias package name and blank identifier like the above.
To check this, modify the main.go
file of the init function example like the below.
package main
import (
"fmt"
_ "github.com/dev-yakuza/study-golang/package/greeting"
)
func main() {
fmt.Println("Hello")
}
And then, when you execute it, you can see the following result.
# go run main.go
init() 0
Hello
import cycle
In Golang, when different packages import each other, the import is repeated and the import cycle
error occurs.
To check this, create the greeting/greeting.go
file and modify it like the below.
package greeting
import (
"fmt"
"github.com/dev-yakuza/study-golang/package/name"
)
func Print() {
fmt.Println("Hello, ", name.Name)
}
And the, execute the following command to create the greeting
module.
# cd greeting
go mod init github.com/dev-yakuza/study-golang/package/greeting
Next, to make the import cycle
error, create the name/name.go
file on the same location with the greeting
folder and modify it like the below.
package name
import (
"github.com/dev-yakuza/study-golang/package/greeting"
)
var Name = "John"
func print() {
greeting.Print()
}
Next, execute the following command to make the name
package.
# cd name
go mod init github.com/dev-yakuza/study-golang/package/name
To connect each packages, execute the following command.
cd greeting
go mod edit -replace github.com/dev-yakuza/study-golang/package/name=../name
go mod tidy
cd ..
cd name
go mod edit -replace github.com/dev-yakuza/study-golang/package/greeting=../greeting
go mod tidy
And then, when you open the name.go
file and check the greeting
package import location, you can see the import cycle not allowed
error like the below.
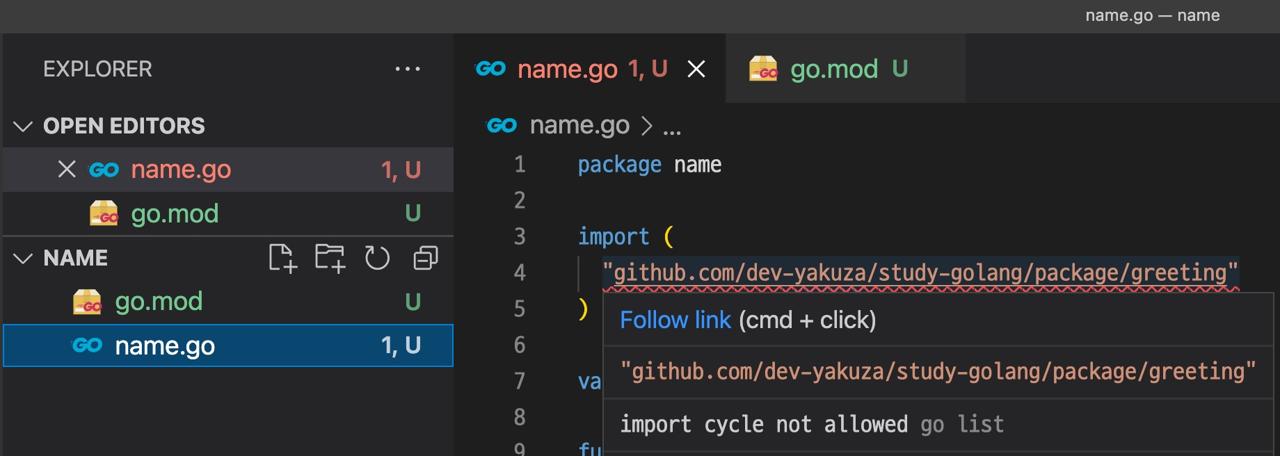
Completed
Done! we’ve seen what Package is and how to use the pcakage in Golang. From now, you can share the package to other programmers, and also you can use the public package to make a program.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.