Contents
Outline
When creating a Pull request
in GitHub
, I was manually setting the creator of the Pull request
to Assignees
as follows.
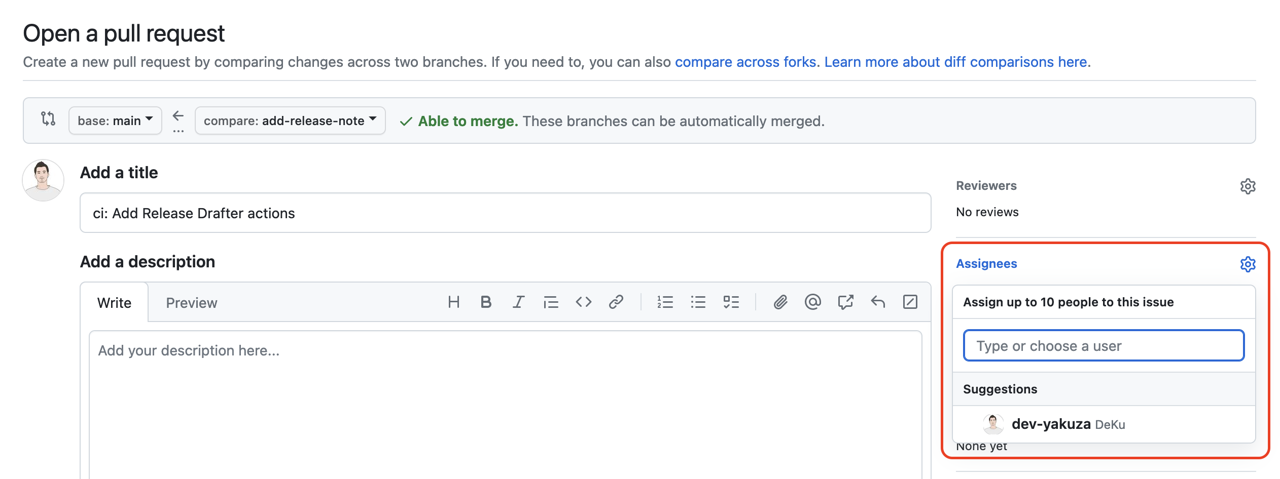
Since the Assignees
of Pull request
is ultimately the person who created the Pull request
, I think it would be good to automatically set the person who created the Pull request
to Assignees
every time a Pull request
is created.
In this blog post, I will introduce how to use GitHub Actions
to automatically set the person who created the Pull request
to Assignees
of the Pull request
.
Create GitHub Actions
Then let’s create a GitHub Actions
that automatically sets the person who created the Pull request
to Assignees
of the Pull request
. Create the .github/workflows/set_assignees.yml
file and modify it as follows.
name: Set assigness
on:
pull_request:
types:
- opened
jobs:
set-assignees:
name: Set assignees
runs-on: ubuntu-latest
timeout-minutes: 1
steps:
- name: Set assignees
run: |
OWNER="$"
REPOSITORY="$"
TOKEN="$"
PULL_REQUEST_NUMBER="$"
ASSIGNEES=$(curl -s \
"https://api.github.com/repos/$OWNER/$REPOSITORY/issues/$PULL_REQUEST_NUMBER" | \
jq --raw-output '.assignees // [] | .[].login')
if [ -z "$ASSIGNEES" ]; then
ASSIGNEE=$
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: token $TOKEN" \
-d "{ \"assignees\": \"${ASSIGNEE}\" }" \
https://api.github.com/repos/$REPOSITORY/issues/$PULL_REQUEST_NUMBER/assignees
fi
I made it to use the API
provided by GitHub
to set the Pull request
creator (github.actor
) to the Assignees
of the Pull request
.
- Official document: List assignees
- Official document: Add assignees to an issue
Dependabot-specific GitHub Actions
I use Dependabot in my personal project. I wanted to set the project Owner
to Assignees
for the Pull request
created by Dependabot
.
For this, I modified the GitHub Actions
as follows.
name: Set assigness
on:
pull_request:
types:
- opened
jobs:
set-assignees:
name: Set assignees
runs-on: ubuntu-latest
timeout-minutes: 1
steps:
- name: Set assignees
run: |
OWNER="$"
REPOSITORY="$"
TOKEN="$"
PULL_REQUEST_NUMBER="$"
ASSIGNEES=$(curl -s \
"https://api.github.com/repos/$OWNER/$REPOSITORY/issues/$PULL_REQUEST_NUMBER" | \
jq --raw-output '.assignees // [] | .[].login')
if [ -z "$ASSIGNEES" ]; then
ASSIGNEE=$
BRANCH_NAME=$
if [[ "${BRANCH_NAME}" == "dependabot/"* ]]; then
ASSIGNEE=$OWNER
fi
curl -X POST \
-H "Content-Type: application/json" \
-H "Authorization: token $TOKEN" \
-d "{ \"assignees\": \"${ASSIGNEE}\" }" \
https://api.github.com/repos/$REPOSITORY/issues/$PULL_REQUEST_NUMBER/assignees
fi
I added dependabot/
to the branch
name and set Assignees
to github.repository_owner
as follows.
BRANCH_NAME=$
if [[ "${BRANCH_NAME}" == "dependabot/"* ]]; then
ASSIGNEE=$OWNER
fi
However this GitHub Actions
did not work properly in Pull request
from Dependabot
. For security reasons, Dependabot
’s Pull request
cannot use any secrets
and can only perform Readonly
operations, so an error occurred.
To fix this, you need to use pull_request_target
instead of pull_request
in on
.
- GitHub Actions: Workflows triggered by Dependabot PRs will run with read-only permissions
- Keeping your GitHub Actions and workflows secure Part 1: Preventing pwn requests
name: Set assigness
on:
pull_request_target:
types:
- opened
actions/github-script
GitHub
provides actions/github-script
that allows you to write more readable code.
By using actions/github-script
, you can modify it as follows.
name: Set assignees
on:
pull_request_target:
types:
- opened
jobs:
set-assignees:
name: Set assignees
runs-on: ubuntu-latest
timeout-minutes: 1
steps:
- uses: actions/checkout@v4
- name: Set assignees
uses: actions/github-script@v6
with:
github-token: $
script: |
const { owner, repo } = context.repo;
const prNumber = context.payload.pull_request.number;
const response = await github.rest.issues.get({
owner,
repo,
issue_number: prNumber,
})
const { assignees } = response.data;
if (assignees.length === 0) {
let assignee = context.actor;
const branchName = context.payload.pull_request.head.ref;
if (branchName.startsWith('dependabot/')) {
assignee = owner;
}
await github.rest.issues.addAssignees({
owner: owner,
repo: repo,
issue_number: prNumber,
assignees: [assignee]
});
}
Completed
Done! We’ve seen how to use GitHub Actions
to automatically set the person who created the Pull request
to Assignees
of the Pull request
. If you are manually setting the person who created the Pull request
to Assignees
every time you create a Pull request
, try using this GitHub Actions
to automate it.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.